Go (Golang): The Unsung Hero In The SEO’s Toolkit
Learn how Go compares to Python and JavaScript, and elevate your SEO automation and coding expertise with this guide. The post Go (Golang): The Unsung Hero In The SEO’s Toolkit appeared first on Search Engine Journal.
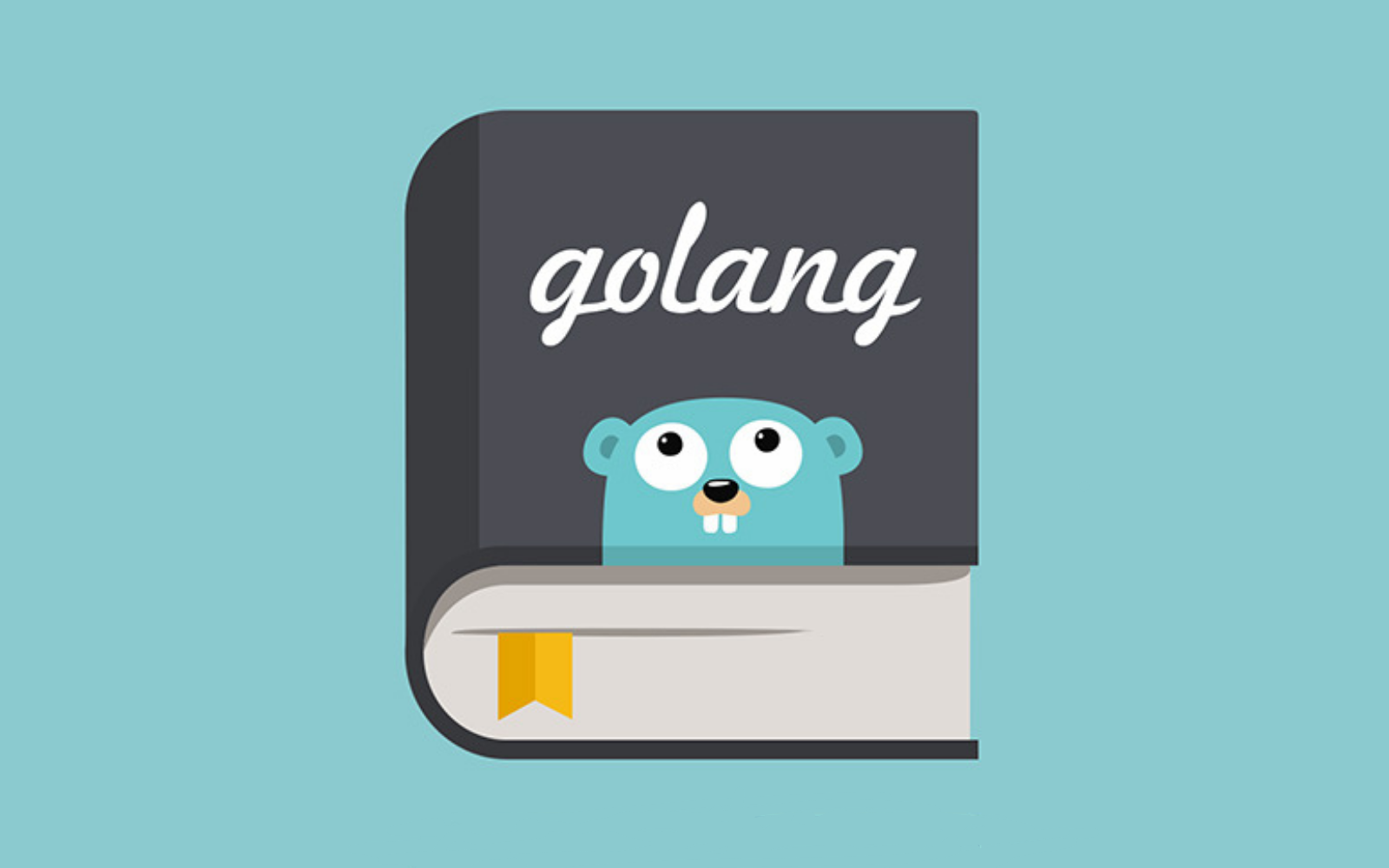
Go is a young language compared to a language like Python, born in 2007.
It has incredible features such as fast execution speed compared to interpreted languages.
It excels in handling concurrency, making it one of the best choices among other languages.
It is easy to scale and has a great community supporting it.
As a technical SEO/SEO developer who develops various tools for automating the SEO process, one of the key points is being fast and efficient in developing and shipping code without complexity.
In these situations, Go (Golang) shines as bright as the sun.
SEO Automation
When it comes to SEO automation, there are many ways to structure the whole project.
You can write your entire app in a single language and package, or you can split your app into microservices/serverless functions and write each service using the language that performs best for it.
If you are working on a simple automation task, this isn’t your concern.
However, as the project grows, you may encounter productivity bottlenecks as an SEO developer.
Who Is An SEO Developer?
When you search to know more about SEO developers, you’ll find some sources that refer to SEO developers as front-end engineers who know about SEO.
However, we know that in an ideal website structure, the app front-end only serves as a shell for the app, not the data.
A back-end engineer is responsible for retrieving the data and returning it to the app’s front end.
The front-end is responsible for creating components with SEO in mind, such as headings, setting titles, meta descriptions, etc.
Furthermore, there are technical SEO experts who also code.
With all these statements in mind, some of us are SEO developers.
Go, JavaScript, and Python: Choose The Right Hammer Based On The Nail
As SEO pros, most of us use Python as the primary language for coding.
With Python, we can perform data analysis on our data, create charts, code web applications using frameworks like Flask, and instantly get a package that crawls the entire site for us, etc. Python is our Swiss Army knife that allows us to do anything.
But what if a new language could help us improve our productivity in some of these tasks?
There are different ways to compare these languages, including their type system, how they handle concurrency, etc.
Before anything else, I assume you are an SEO developer who knows Python/JS.
If you are new to coding as an SEO, Python is the better choice for you in most cases, rather than learning JS or Go.
Below is my preference for the task: each language can better serve me as an SEO professional:
Python: Analyzing data and performing ML-related tasks. JavaScript: Writing Apps Script, working with Google Tag Manager as an advanced user. Interested in front-end development. Go (Golang): Developing web applications. Interested in back-end development.Go Facts
Let’s become more familiar with the Go language.
Go History
If you are reading this section, you’ve decided to learn Go as your new coding language.
Let’s briefly look at Go’s history.
Go was developed by Robert Griesemer, Rob Pike, and Ken Thompson at Google in 2007 and launched as an open-source programming language in 2009.
It’s interesting to know that it was inspired by Python (created in the late 1980s) for its simplicity.
Go Is A Compiled Language
Besides the fact that compiled languages are faster in execution than interpreted languages, when I code in Python and want to execute it on another machine, I find myself struggling to obtain the packages, resolve package conflicts, and so on.
However, with Go, I can easily build the code on my non-Linux machine for the Linux machine where I intend to run the code.
Then, I use the “scp” command-line tool to copy the executable to the Linux machine.
Go Has A Fabulous Standard Library
One of the amazing aspects of Go is that you can explore its standard library.
If you want to make a request, instead of downloading the “requests” package in Python, you can use the built-in package.
Similarly, if you want to create a web server, you can do it without installing any additional packages. In many cases, you only need to consult the Go standard library to find a solution for your problem.
Go Is Fast
When we say Go is fast, we can assess its speed from various perspectives. Here are some of these aspects:
Because of its simplicity, you can have a nice and fast development experience. It’s an effective garbage collection that manages the memory for you. Concurrency is one of the things that Go is famous for, and it’s easy to set up (unlike Python). Since Go is a compiled language, you get relatively faster code execution compared to interpreted languages.What Are The Tools For Coding Go?
There are several options for coding in Go. Below, you can see a list of these tools:
Visual Studio Code (VS Code) – Free. GoLand – Paid. Vim/Neovim – Free.Personally, I code in GoLand, but with the IdeaVim plugin that brings Vim motions to my IDE and makes my life easier.
If you want to use VS Code, install the official Go plugin for it. If you prefer Vim/Neovim, don’t forget to install Go LSP.
How To Install Go
You can easily follow the instructions the Go website provides to install Go based on your operating system.
How To Write Hello World In Go
Let’s GO.
After installing the Go language on your computer and verifying that it’s installed, create a folder wherever you want and name it “hello-go.”
Then, on your terminal or Windows Subsystem for Linux, use the “cd” command to navigate to the folder you created.
Since you have installed Go, you can now access the Go command line on your machine.
In your command line, execute the “go mod init hello” command.
This command will create a “go.mod” file, which declares the module name, the required Go version, package dependencies, and more.
If you don’t understand it yet, don’t worry – take the time to learn it without stopping at this moment. If you’re familiar with Poetry for your Python projects, they have some similarities.
Now let’s create a file to write our code in and name it “hello.go.” If you are creating the file using your terminal, you can run the command “touch hello.go“.
Let’s open our text editor/IDE to write our first Go code. Below, you can see the code, and then I will explain it to you.
package main
import "fmt"
func main() {
fmt.Println("Hello GO!")
}
There Are Different Elements To Consider About The Above Code
Package name: We named it “main” to indicate that this is our main package in Go. Import statement: Similar to Python, we imported the “fmt” package from the standard library, which is used for formatted I/O. Main function: This serves as the entry point for Go to run our program.Maybe now you are asking yourself, “Alireza said Go is easy, and what are the hacks above? Python is simpler, etc.”
There are typically some differences between Python and Go, but at this moment, let’s consider that we write our functions outside the main function and then call them inside the main function.
When Go wants to run our program, it knows it must run the main function. So, if there is a call to another function, it goes and runs it.
If you are familiar with languages like C, the above code will be much more familiar to you.
How To Run/Build Our Program In Go
After coding our program, we need to run it to see “Hello GO!” as the output. To do this, we run the command “go run hello.go” in the command line.
As you can see, we have our output.
As I mentioned, Go compiles our code and is not similar to Python, which uses an interpreter. Therefore, we can obtain an executable file and run it!
When we use the “go run” command, it creates the executable file on the fly and runs it without saving it. However, by executing the “go build hello.go” command, we obtain our executable file as output with the same name as the file we passed to “go build.”
After running “go build hello.go” we should have a “hello” file as the output, which is an executable file. We can run it from the terminal using the “./hello” command.
Python Code Equivalent In Go Code
Now that we know the basics let’s look at creating a variable, a for loop, making an HTTP request, etc.
Below, I will write both Python code (which I assume you are familiar with) and the equivalent code in Go for a better understanding.
The project “Golang for Node.js Developers” inspired me to write in this way. I also created a project called “Go for Python Developers” with as many examples as possible for me, so don’t miss it.
Let’s GO!
Variables
Python:
mutable_variable = 2
CONST_VARIABLE = 3.14 # There isn't a way to define a constant variable in Python
a, b = 1, "one" # Declaring two mutable variables at once
Go:
var mutableVariable int = 2
const ConstVariable float = 3.14
var a, b = 1, "one" // Go automatically assigns types to each variable (type inferred), so you can't change them later.
Besides that, the above example shows you how you can create variables in Go.
You can also see the different ways of naming variables in Go and Python, as well as the various ways of leaving a comment.
Data Types
Python:
string_var = "Hello Python!"
integer_var = 2
float_var = 3.14
boolean_var = True
Go:
var string_var string = "Hello Go!"
var integer_var int = 2
var float_var float = 3.14
var boolean_var bool = true
For loops
Python:
i = 10
for i in range(10):
print(i)
Go:
// Initial; condition; after loop
for i := 0; i < 10; i++ { // Using the shorthand syntax of declaring a variable in Go (mutableVar := the value)
fmt.Println(i)
}
While loop
Python:
counter = 0
while counter < 5:
print(counter)
counter += 1
Go:
var counter int = 0
for counter < 5 {
fmt.Println(counter)
counter += 1
}
If/Else:
Python
age = 25if age >= 13 and age <= 19:
print("Teenager")
elif age >= 20 and age <= 29:
print("Young adult")
elif age >= 30 and age <= 39:
print("Adult")
else:
print("Other")
Go:
var age int = 25
if age >= 13 && age <= 19 {
fmt.Println("Teenager")
} else if age >= 20 && age <= 29 {
fmt.Println("Young adult")
} else if age >= 30 && age <= 39 {
fmt.Println("Adult")
} else {
fmt.Println("Other")
}
Array/Slice
In Python, we are familiar with lists whose size is dynamic. In Go, there are two different concepts for Python lists. The first is an Array, which has a fixed size, and the second is Slices, which is dynamically sized.
Another important thing about Arrays/Slices in Go is that we must define the type of elements we want to store in our Array/Slice. In other words, you can have a slice of strings, not a slice of both strings and integers.
Python:
mix_list = [False, 1, "two"]
Go:
var boolArray [3]bool = [3]bool{false, true, true} // var variableName [array size]type of array elements
var stringArray [3]string = [3]string{"zero", "one", "two"}
var intArray [3]int = [3]int{0, 1, 2}
var boolSlice []bool = []bool{false} // var variableName []type of slice elements
var stringSlice []string = []string{"zero", "one"}
var intSlice []int = []int{0, 1, 2}
For loops Over Arrays/Slices
Python:
mix_list = [False, 1, "two"]
for item in mix_list:
print(item)
Go:
var intSlice []int = []int{0, 1, 2}
for index, value := range intSlice {
fmt.Println(index, value)
}
Maps
Consider Map as the dictionary that we have in Python. Similar to an Array/Slice, you must declare the type of the key and the type of the values.
Python:
the_dictionary = {"hi": 1, "bye": False}
print(the_dictionary["hi"])
Go:
var theMap map[string]int = map[string]int{"hi": 1, "bye": 0}
fmt.Println(theMap["hi"])
HTTP Get Request
Python:
import requests
response = requests.get("https://example.com/")
print(response.content)
Go:
import (
"fmt"
"io"
"log"
"net/http"
)
resp, err := http.Get("https://example.com/")
if err != nil {
log.Println(err)
}
defer resp.Body.Close()
body, err := io.ReadAll(resp.Body)
fmt.Println(string(body))
Conclusion
If you are interested in learning Go, you can check the Go by Example website (I considered its hierarchy of examples for this article) and the Go Tour.
Hope you enjoy coding in Go, and happy learning!
More resources:
Website Development: In-Depth Guide For Beginners Streamlit Tutorial For SEOs: How To Create A UI For Your Python App An Introduction To Python & Machine Learning For Technical SEOFeatured Image: ShadeDesign/Shutterstock